Today’s post will be short. I went and saw The Batman tonight, it was really good.
After getting home I wanted to try and add something quick to the game, so I decided I wanted to add a simple particle trail behind the ball.
This would be super simple in Unity, however, as far as I could find in Godot it gets a bit more simple. Shout out to picster on youtube, who made a great tutorial on this very subject that I based my trail on.
However, his full tutorial was way more complicated and pretty than I either had time to implement or really needed, but my trail is built off the same principle.
I made a trail scene, with the base node being a Line2D, the curve is similar, as is the gradient, but my texture is just a solid block, rather than the smokey texture he used for his smoke trails.
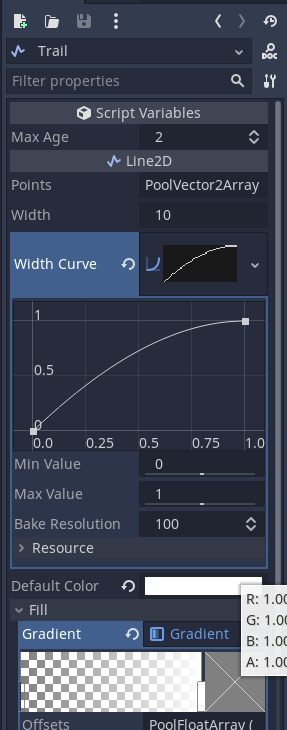
My script is also a lot more bare bones than his. Also, since my ball is moving constantly and I didn’t want to have an endless stretching trail behind it, I made a change to where I delete the old points after they had been around long enough. My full trail script is below.
extends Line2D
export var max_age = 2
var age_of_points = [0.0]
func _ready():
set_as_toplevel(true)
func _process(delta):
if age_of_points[0] > max_age:
age_of_points.remove(0)
.remove_point(0)
for p in range(get_point_count()):
age_of_points[p] += 5 * delta
func add_point(point_pos:Vector2, at_pos = -1):
age_of_points.append(0.0)
.add_point(point_pos, at_pos)
func _on_Decay_tween_all_completed():
queue_free()
I also made a small change to the ball scene, linking in the trail under it, and adding a call to app_point in the trail as well.
extends KinematicBody2D
export (int) var speed = 300
export (int) var increase_by = 50
var current_speed
var velocity = Vector2()
onready var trail = $Trail
func _init():
velocity.x = 1
velocity.y = 1
current_speed = speed
func _process(delta):
trail.add_point(global_position)
var move_by = velocity.normalized() * current_speed
var coll = move_and_collide(move_by * delta)
if (coll != null):
var hit = coll.get_collider()
if (hit.is_class("KinematicBody2D")):
$PaddleHitEffect.play()
handle_paddle_reflect(self, hit)
current_speed += increase_by
else:
$WallHitEffect.play()
velocity = velocity.bounce(coll.normal)
func handle_paddle_reflect(ball : KinematicBody2D, paddle: KinematicBody2D):
var is_pos = velocity.y > 0
if (velocity.y == 0):
is_pos = ball.position.y > paddle.position.y
var hit_from_center = abs(ball.position.y - paddle.position.y)
if (hit_from_center <= 5):
velocity.y = 0
elif (hit_from_center <= 25):
velocity.y = 1 if is_pos else -1
elif (hit_from_center <= 75):
velocity.y = 1.5 if is_pos else -1.5
else:
velocity.y = 2 if is_pos else -2
And with that I have a simple trail added to the ball as it bounces around.
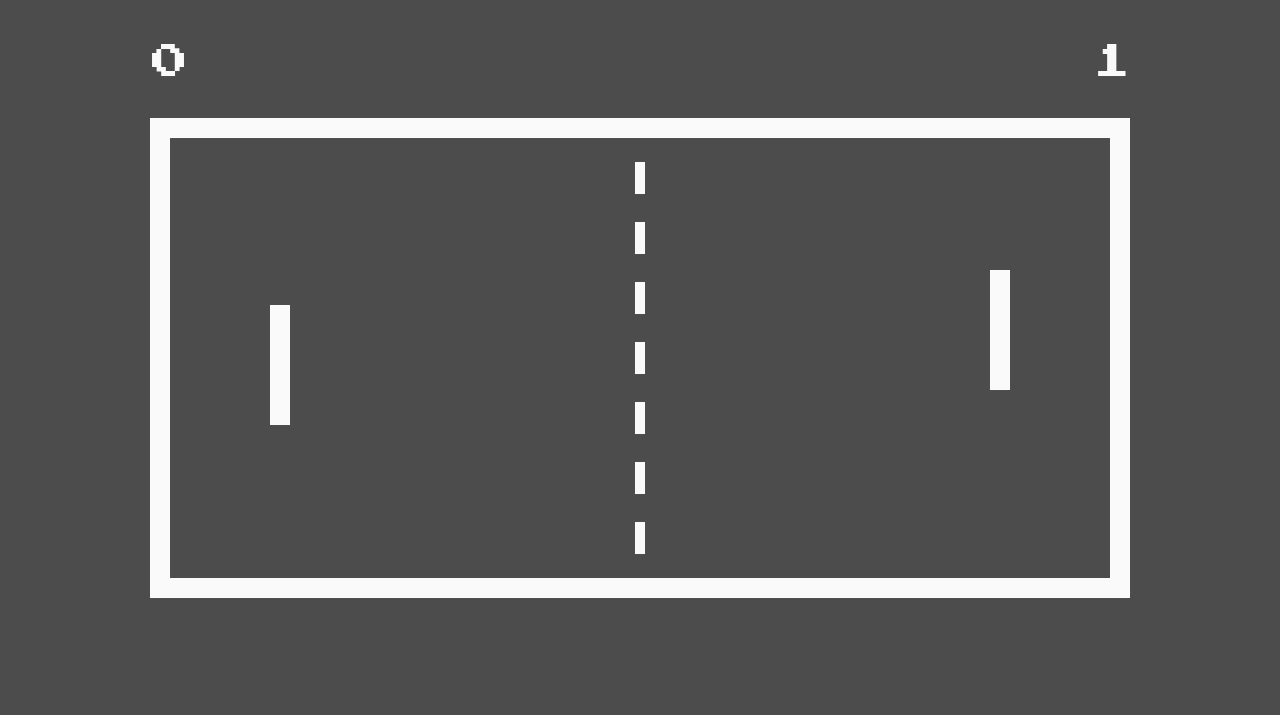
-Ike